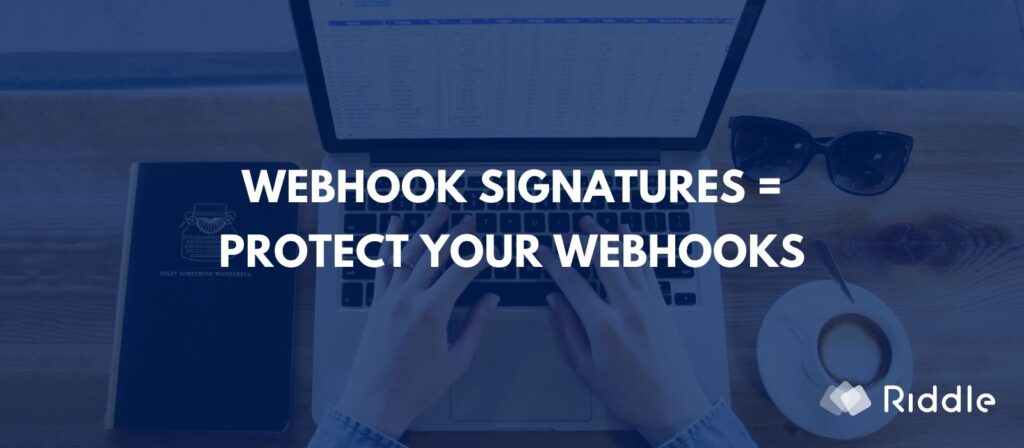
Riddle’s webhooks are a great way to process all the data generated by a Riddle on your own servers. But you need to be aware of mischievous users who might want to misuse the webhook to send altered data to your endpoint.
Fortunately – we’ve got you covered.
Quiz webhook signatures – use case
Just imagine the following scenario:
- You run a quiz on your site and you want to make sure that only entries from the official riddle.com servers can be sent to your your own database.
- We’ve got you covered – you can use our nifty webhook signatures to secure the data transfer from riddle to your database.
- With these signatures you can be sure that the data’s origin is riddle.com + the data was not tampered with.
- Without validation tech-savvy users could simply change the data that’s being sent to your site and could change their results that way.
Signature keys per project and endpoint URL
Riddle generates a new and unique key based on these conditions:
- Each project has a unique signature
- Each endpoint has a unique signature
This means that if you are using the same endpoint in all your Riddles in a project, the signature will always be the same. If you use multiple endpoints for your Riddles in a project each of these endpoints will have a unique signature in your project.
If you use the same endpoint in different projects, the signature will be different in each of the projects.
Finding your webhook signature
As the signature can be different depending on the endpoint you are using, we are displaying it when you add a new endpoint. When an endpoint is enabled we send some test data to your endpoint and display the key upon a successful connection. Please make a copy of the signature and store it in a safe place. To view it again, you can test the webhook in your Riddle at any time.
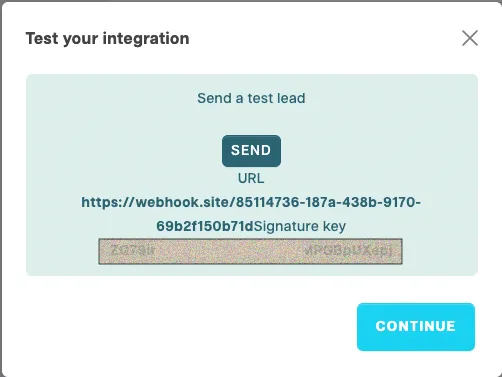
Sample code
Our webhook signature uses a public / private key combination to secure a webhook. By adding the secret key from the Riddle to your code you will be able to verify the public key in the webhook payload. The sample code below will fail with an error message if the public key cannot be verified using the secret key. If you are curious to learn more about public / private key encryption, please check out this Wikipedia article.
<?php
$signatureKey = 'put the key here'; // found under save leads -> webhook -> test webhook
$receivedSignature = @$_SERVER['HTTP_X_RIDDLE_SIGNATURE'] ?
$_SERVER['HTTP_X_RIDDLE_SIGNATURE'] : null;
if (null === $receivedSignature) {
\http_response_code(403);
die('Access denied - did not receive any signature.');
}
$payload = \file_get_contents('php://input');
$generatedSignature = \hash_hmac('sha256', $payload, $signatureKey);
if (!\hash_equals($generatedSignature,$receivedSignature)) { // the signature does not match - reject this webhook
// \http_response_code(403);
die('Access denied - invalid signature. received: ' . $receivedSignature . ', generated: ' . $generatedSignature);
}
$payload = \json_decode($webhookContent, true);
// now work with $payload['data']['riddle'] ...
// you could use the line below to write the payload data into a text file
//\file_put_contents(__DIR__.'/payload.json', $payload);
Please make sure to also check out our article on how to work with webhooks here.
Riddle 1.0 (legacy): add webhook signatures
If you are using our old and deprecated version of Riddle, please follow these instructions to find and use your key.
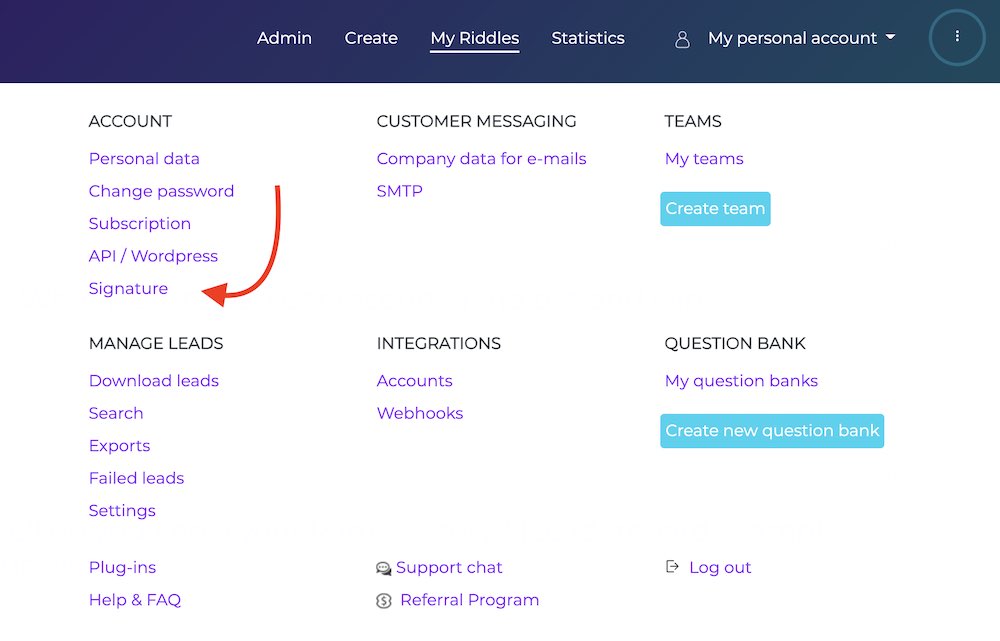
To help you getting started we’ve created the following snippet – please make sure to replace the key below with your generated secret signature key.
IMPORTANT: please make sure to use PHP version 5.6 or higher
<?php
$privateKey = 'YOUR SECRET KEY'; // found under Account > Signature
$receivedSignature = $_SERVER['HTTP_X_RIDDLE_SIGNATURE_2'] ?
$_SERVER['HTTP_X_RIDDLE_SIGNATURE_2'] : null;
if (!$receivedSignature) {
\http_response_code(403);
die('Access denied - did not receive any signature.');
}
$data = \file_get_contents('php://input');
$generatedSignature = \hash_hmac('sha256', $data, $privateKey);
if (!\hash_equals($generatedSignature,$receivedSignature)) { // the signature does not match - reject this webhook
\http_response_code(403);
die('Access denied - invalid signature. received: ' . $receivedSignature . ', generated: ' . $generatedSignature);
}
// do anything - the signature matches and you can be sure that the data is from riddle.com
echo 'SIGNATURE CHECK SUCCEEDED!';
Feel free to implement this kind of validation with any other programming language.
Any questions about webhook signatures?
If you run into any problems, feel free to ask us using support chat (we’re lightning fast to reply) or email (hello@riddle.com).
Seriously, we’ve been big quiz (and customer support geeks) since 2014 – we all love to help.