Our API version 3.0 is now (March 2023) available. The full API documentation can be found here.
To help you get started, you can either use Postman or the sample PHP script below.
When using Postman, please add the authentication parameters to the Headers as shown in the screenshot below. The token needs to be preceded with the string “Bearer”
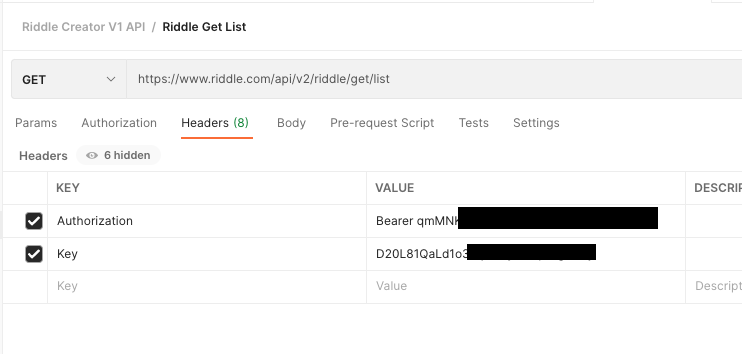
It wraps all the functions of our API and allows you to use them with only 2 lines of code.
Please make sure that you inserted your token & API key which can be found either on https://www.riddle.com/creator/account/api or on the edit site of your chosen team.
Keep also in mind how our API responds (https://www.riddle.com/help/riddle-v1/api/v2/#section/Responses-from-the-API): our snippet returns the whole response and not just the body itself – feel free to change this snippet.
class ApiConnector
{
private $token, $key;
private $url = 'https://www.riddle.com/api/v2';
public function __construct($token, $key) {
$this->token = $token;
$this->key = $key;
}
โ
protected function request($path, $params = null, $method = 'GET', $jsonDecode = true)
{
$url = $this->url . $path;
$ch = curl_init();
curl_setopt($ch, CURLOPT_HTTPHEADER, ["Authorization: Bearer $this->token", "Key: $this->key"]);
โ
if ($params) {
$this->_prepareArrayValues($params);
if ('POST' === $method) {
curl_setopt($ch, CURLOPT_POSTFIELDS, $params);
}
if ('GET' === $method) {
$url .= '?' . http_build_query($params);
}
}
โ
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
โ
return $jsonDecode ? json_decode($response) : $response;
}
โ
private function _prepareArrayValues(&$params)
{
foreach ($params as $_key => $_value) {
if (is_array($_value)) {
$params[$_key] = implode(',', $_value);
}
}
}
โ
}
โ
class Api extends ApiConnector
{
public function getRiddleTypes()
{
return $this->request('/riddle/get/types');
}
โ
public function getRiddleList($params = null)
{
return $this->request('/riddle/get/list', $params);
}
โ
public function getRiddleEmbedCode($riddleId)
{
return $this->request('/riddle/get/embed-code', ['riddleId' => $riddleId], 'GET', false);
}
โ
public function getTeams()
{
return $this->request('/team/get/list');
}
โ
public function getTags($params = null)
{
return $this->request('/tag/get/list', $params);
}
โ
public function getAuthors($teamId = null)
{
return $this->request('/author/get/list', ['teamId' => $teamId]);
}
โ
}
โ
$api = new Api('Insert your Bearer here', 'Insert your API key here');
var_dump($api->getRiddleTypes()); // simple test
Have any questions about our API? Please give us a shout on support chat or at hello@riddle.com – we’re super fast to respond to every message.
(Our entire team, from our CEO Boris on down, race each other to answer messages first.)